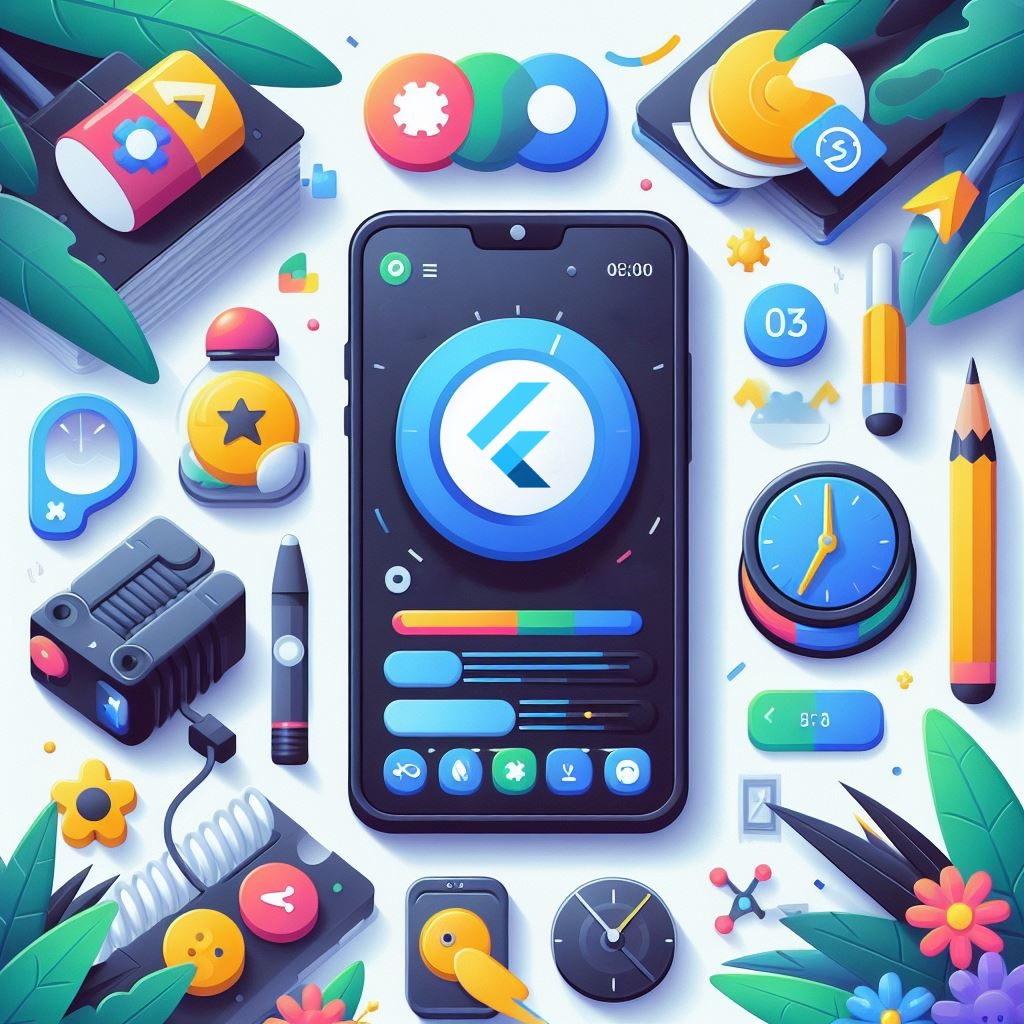
Flutter is a versatile open-source framework that helps craft natively compiled applications for mobile, web, and desktop, everything from a singular codebase. What makes Flutter truly stand out is its reliance on widgets, which are the building blocks of its user interface. These widgets aren't just chunks of code; they're dynamic, customizable elements that streamline the development process and ensure a consistent and visually appealing user experience across different platforms.
Table of contents
- Widget Basics
- Commonly Used Widgets
- Widget Trees
- Advanced Widgets
- Theming and Styling
- State Management
- Custom Widgets
- Hot Reload and Instant Feedback
- Conclusion
Widget basics
In Flutter, widgets are the elemental components that make up your app's user interface. Think of them as the Lego bricks of your application. They range from basic structural elements to complex interactive pieces, all serving specific purposes in crafting the overall user experience.
Now, let's dive into the widget taxonomy. There are two main types: stateless and stateful widgets.
- Stateless Widgets: These are immutable, meaning their properties (attributes) cannot change once they're set. Stateless widgets are ideal for static content or elements that don't evolve based on user interactions. Picture a button with a fixed label; no matter how many times you press it, the label remains the same.
- Stateful Widgets: On the flip side, stateful widgets are mutable and dynamic. They can be altered during runtime, adapting to user input or changes in the app's internal state. Think of a checkbox that can toggle between checked and unchecked states.
Now, let's talk about what makes all this possible: the build
method. In Flutter, every widget has a build
method responsible for constructing the widget's subtree. The build
method is called whenever the widget needs to be rendered or re-rendered, allowing for dynamic updates in response to changes in the app's state or user input.
Commonly used widgets
Let's talk about some of Flutter's commonly used widgets that usually take part in the building process of an application:
- Container: This one's like the superhero of layout and styling. If you need a box to put stuff in that you might want to customize, the
Container
widget is what you should go with. It's versatile, customizable, and basically the go-to for arranging and decorating your widgets. - Row and Column: Their main functionality is organizing widgets horizontally and vertically.
Row
lines up widgets side by side, whileColumn
stacks them up like a tower of digital blocks. - ListView:
ListView
lets you create lists of widgets that users can scroll through effortlessly. It's your go-to for making long lists of items, whether it's a to-do list or a gallery of cat pictures. - AppBar:
AppBar
is the top-level bar in your app, providing a space for titles, icons, and all things navigation. - Scaffold:
Scaffold
is the backbone, the structure that holds everything together. Scaffold sets the stage for your app's visual interface, giving you a place to put your app bar, body content, and all the widgets of the application.
Widget trees
Think of a widget tree as a family tree for your user interface. At the root, you have the main widget, which could be something like the entire application window. Then, as you go down the tree, each widget represents a different part of the UI, like buttons, text boxes, images, etc.
Now, nesting widgets is like building with Lego blocks. You start with simple ones, like a basic button or text field, and then you stack them together to create more complex structures. For instance, you might have a widget for a chat window that contains nested widgets for the chat history, input field, and send button.
Efficiency is key. Imagine you're organizing your closet. In an ideal case, you wouldn't want to dig through a pile of clothes to find your favourite shirt. Similarly, in a widget tree, structure and order matter. Group related widgets together, use containers to hold them and keep things tidy. It not only makes your code more readable but also helps in debugging and maintaining your UI.
Advanced Widgets
FutureBuilder: Handling asynchronous operations
FutureBuilder
is a widget designed for managing asynchronous operations, particularly Futures. It's like having a crystal ball to predict when something will be ready in the future. Imagine you're baking a cake. You don't want to just stand there staring at the oven, because you have other tasks.FutureBuilder
lets your app do other things while waiting for a future (like fetching data) to complete. Once the future resolves,FutureBuilder
automatically rebuilds the UI with the fetched data.StreamBuilder: Managing streams of data
StreamBuilder
, on the other hand, is designed for managing streams of data. It allows you to react to changes in a stream and update your UI in real-time. Think of a live chat app whereStreamBuilder
listens to the stream of incoming messages. When a new message arrives, it triggers a rebuild, updating the chat UI dynamically.The role of keys in widget management
In widget management, keys play a crucial role. Keys are like social security numbers for widgets, providing a unique identity to each widget. They help Flutter keep track of widgets, especially in scenarios where widgets might be moving or changing. For example, if you have a list of items and you want to update them, keys ensure that Flutter knows which item is which, preventing unnecessary rebuilding and enhancing performance.Performance optimization techniques
When it comes to optimizing performance in Flutter, there are specific techniques to ensure your app runs smoothly.ListView.builder()
is perfect for handling long lists. It creates widgets only for the items visible on the screen, saving resources. Additionally, const Constructors can be used to optimize performance. If a widget doesn't change, making it const informs Flutter that it's constant, preventing unnecessary recreation and contributing to a well-optimized app, even when dealing with a large codebase.
Theming and styling
Theming and styling in Flutter play an important role in creating visually appealing and consistent user interfaces across applications. Flutter's approach to theming revolves around the use of widgets and a robust styling system. At the core of this system is the concept of the ThemeData
class, which encapsulates the visual design elements of an application. It includes attributes like the primary and accent colours, typography, and various other settings that define the overall look and feel.
One of the key widgets contributing to theming is the MaterialApp
widget, which is often the root of a Flutter application. MaterialApp
allows you to define a theme that gets inherited by its child widgets, ensuring a cohesive design language. The Scaffold
widget provides a visual structure for the application and incorporates theming through its appBar, body, and other elements.
Flutter's flexibility in theming extends to individual widgets as well. Widgets like Text
, AppBar
, and FloatingActionButton
allows customize their appearance through style attributes, aligning with the global theme or deviating as needed. With this approach, it’s very easy to achieve both consistency and unique designs within the applications. In addition to predefined themes, Flutter also supports the creation of custom themes tailored to specific brand identities or design preferences.
State management
Now, imagine you have a bunch of widgets in your Flutter app, and they all need to share some information or keep track of certain changes. How do you handle that? Well, that's where InheritedWidget
comes into play.
InheritedWidget
is like a messenger that carries a piece of data and shares it with all the widgets below it in the widget tree. It's inherited by the widgets, hence the name. So, if a widget deep down the tree needs to know about a piece of data, it can just inherit it from its ancestors.
Now, let's talk about why effective state management is crucial. Flutter is all about widgets rebuilding and updating to reflect changes. But without proper state management, things can get messy. You might end up with widgets rebuilding unnecessarily or not updating when they should. This can lead to performance issues and a confusing, buggy user experience.
Effective state management ensures that your app stays responsive and efficient, and maintains a clean structure. Flutter provides various state management approaches, and the key is to choose the one that fits your app's needs best. Whether it's using setState
, Provider
, Bloc
, or other state management libraries, the goal is to keep your app's state organized and accessible to the widgets that need it.
Custom widgets
Custom widgets are what add that extra flavour to your Flutter app. They not only make your code more modular and organized but also allow for easy reuse across different parts of your application.
Creating custom widgets involves several steps. Firstly, identify reusable components in your UI, such as buttons, cards, or other widgets used frequently. Once identified, create a Dart class for the component, extending either StatelessWidget
or StatefulWidget
based on whether your widget needs to maintain state. Implement the build
method within your custom widget class to define its structure and appearance. Additionally, make your widget flexible by adding parameters, so that you can customize aspects like text, color, or callbacks.
The role of encapsulation is crucial in creating maintainable code. Encapsulate the internal workings of your widget, exposing only the necessary properties and methods. This practice shields the internals from unintended interference. By encapsulating details, you ensure that changes and improvements to the widget won't break other parts of your application. This approach enhances code reliability and maintainability, making it easier to manage and evolve your Flutter app.
Hot reload and instant feedback
Flutter's hot reload is like having a superpower in your development toolkit. It allows you to make changes to your code and instantly see the results without restarting the entire application. Whether you're tweaking the UI, fixing bugs, or experimenting with new features, hot reload speeds up your development cycle by applying changes on the fly.
The real beauty of hot reload lies in the instant feedback it provides. As a developer, you get to see the impact of your code changes immediately. This rapid feedback loop is a game-changer. It not only accelerates the development process but also boosts creativity. You can iterate, experiment, and fine-tune your app without the frustration of long waiting times.
Imagine tweaking the styling of a button, adjusting the layout, or refining the logic—all while the app is running. The instant feedback loop turns coding into a dynamic and interactive experience. It reduces the cognitive load, allowing you to focus on the task at hand rather than waiting for the code to compile and the app to restart.
Conclusion
In conclusion, Flutter opens up a world of possibilities for developers, providing a seamless and efficient way to create cross-platform applications. The power of widgets, from the basic Container to the advanced FutureBuilder and StreamBuilder, enables the building of dynamic, responsive, and visually stunning user interfaces.
Understanding the importance of efficient widget management, theming, state management, and the creation of custom widgets is crucial in crafting maintainable and attractive Flutter applications. Hot reload adds a dynamic layer to the development process, accelerating iteration and debugging.
If you’d like to get more information about Flutter’s widget catalog you can do that in Flutter’s official documentation.